Arrange, Act and Assert Pattern: The Three A’s of Unit Testing
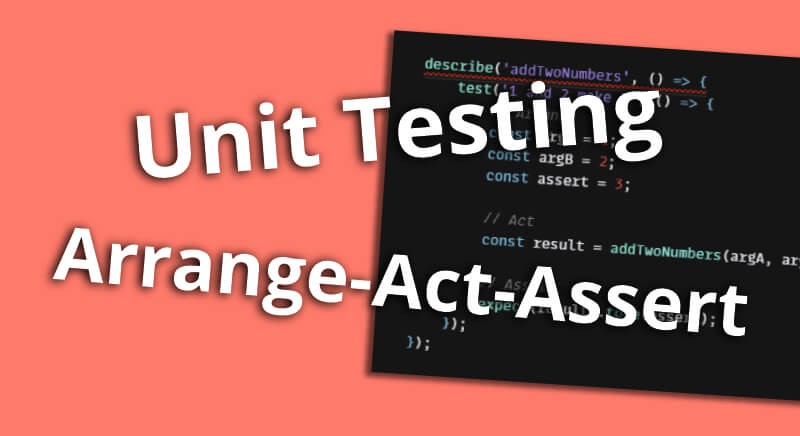
This post contain affiliate links to Udemy courses, meaning when you click the links and make a purchase, I receive a small commission. I only recommend courses that I believe support the content, and it helps maintain the site.
If you’re new to unit testing, the three A’s pattern (Arrange-Act-Assert, or AAA) is a great place to start. Arrange, Act and Assert is a simple pattern that can be applied to any unit test.
What is the Arrange, Act and Assert Pattern?
The Arrange, Act and Assert Pattern is a way of structuring unit tests so that they are easy to read and understand. This pattern is also known as the Given, When, Then pattern. The order is important, and shouldn’t be confused with Act Assert Arrange, or Act Arrange Assert! The steps to the three A’s are:
Arrange: Set up the conditions for your test. This might involve creating objects, setting up variables or anything else that’s required for your test.
Act: This is where you actually execute the code that you are testing.
Assert: Verify that the code you’re testing behaves as expected. This might involve checking the value of a variable, or verifying that a certain method was called.
The Benefits of the Arrange, Act and Assert Pattern
The Arrange, Act and Assert pattern can help you write better unit tests. It is a simple yet effective way to write better unit tests. By breaking down your tests into these three distinct stages, you can more easily identify what needs to be tested and ensure that your tests are comprehensive. There are several benefits to using the AAA pattern for unit testing.
Helps to keep the tests concise and focused
By following a strict structure, tests are easier to write when they are smaller and only look to test a singular thing. The test should only ‘assert’ one outcome. By limiting tests in this way, everything becomes more manageable.
Tests are easier to read and understand
Following the Arrange Act Assert pattern means that tests not only prove that the function works, they demonstrate how the function or component works. As they are more concise, they should show that by changing one argument or environment factor, they perform in a particular way. This should be documented by the test, providing a walk through of the function and what it does.
Debugging can be easier
This pattern can make debugging fair easier because each test is an isolated unit. There should be no external factors the change how the test performs. On top of this, as each test is focused on a singular outcome it can be a lot easier to see exactly what broke. There is some argument that lots of small tests are not worth the time, but testing utility functions in this manner can be beneficial. Team members can change over time and accidently break a function without meaning to. Unit tests can catch this small mistakes.
How to use the Arrange, Act and Assert Pattern
I use the Arrange, Act and Assert Pattern in Jest unit tests, and when using React Testing Library. In this blog I will only cover Jest tests, but the same pattern applies to other situations.
The Function
For the sake of this article, the function that will be tested is very small. It takes two arguments (a and b) and adds them together then returns the result.
const addTwoNumbers = (a, b) => a + b;
From this, we know that the function takes two arguments that can change, and there are no other variables that can change. With this in mind we know what we need to ‘arrange’. The ‘act’ section will be passing the variables into the function and running it, Then the test will ‘assert’ the expected outcome.
The Test
describe('addTwoNumbers', () => {
test('1 and 2 make 3', () => {
// Arrange
const argA = 1;
const argB = 2;
const assert = 3;
// Act
const result = addTwoNumbers(argA, argB);
// Assert
expect(result).toBe(assert);
});
});
And that is it. However this pattern can then be used on far more complex functions – forcing the developer to focus on what is happening, and why.
Arrange-Act-Assert is powerful because it is simple. By keeping the tests short, concise and to the point leads to clean tests. Tests that are easier to write and easier to debug!