How to Mock an ES6 Module Import using Jest
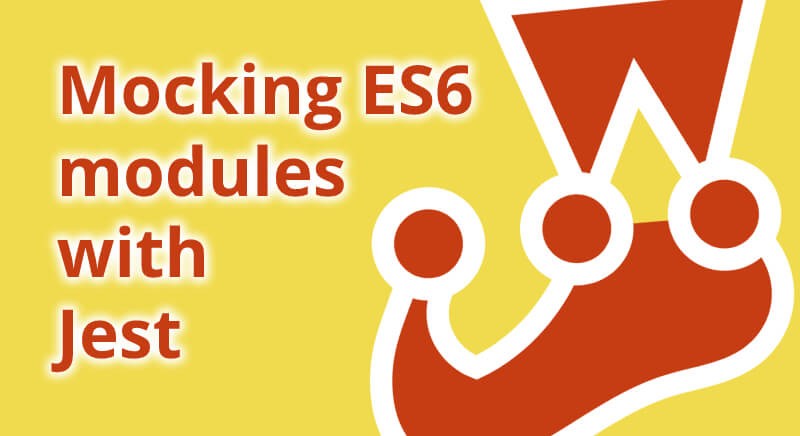
This post contain affiliate links to Udemy courses, meaning when you click the links and make a purchase, I receive a small commission. I only recommend courses that I believe support the content, and it helps maintain the site.
What is an ES6 Module?
ES Modules are the ECMAScript standard for working with modules. Node has used this standard for a while, and browsers have only recently (2016) started using ES6. ES6 functions are the same as regular functions with syntactic sugar. So they can be mocked in the same way!
For a more in-depth look into ES6 Modules take a look at this piece.
How to Mock an ES6 Module
The jest.mock
function is used to mock an ES6 module import with Jest. The initial function would look like:
// ./functionToTest.js
import {
es6ModuleToMock,
es6ModuleToReturnThing
} from './fileWithModuleToMock';
export const functionToTest = () => {
const value = es6ModuleToReturnThing();
es6ModuleToMock(value);
};
And the test file would look like:
import functionToTest from './functionToTest';
import {
es6ModuleToMock,
es6ModuleToReturnThing
} from './fileWithModuleToMock';
jest.mock('./fileWithModuleToMock');
test('example of how to mock an ES6 module', () => {
const mockValue = 'mock-value';
es6ModuleToReturnThing.mockReturnValue(mockValue);
functionToTest();
expect(es6ModuleToMock).toBeCalledWith(mockValue);
});
What we are doing is importing the particular functions that need to be mocked, and then mocking the whole file with Jest. This allows us to work with different functions run different mocks on them.
Take a look at the article on testing with Jest and React Testing Library for a basic overview of unit testing.