Migrating from query-string to URLSearchParams
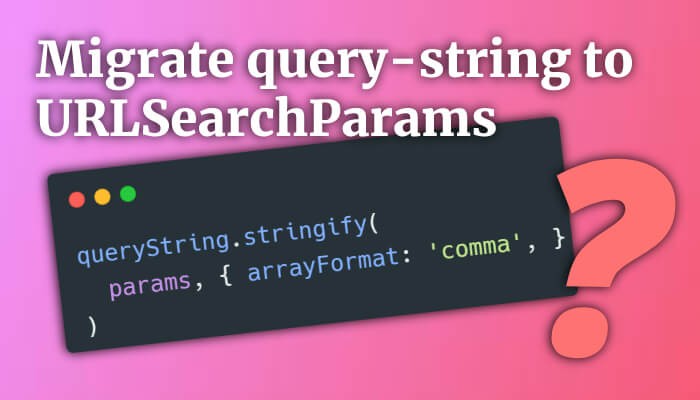
This post contain affiliate links to Udemy courses, meaning when you click the links and make a purchase, I receive a small commission. I only recommend courses that I believe support the content, and it helps maintain the site.
In my quest to remove as many third-party packages as possible from mine and my clients websites, I have been working through each package and checking if there is a native replacement.
One package that was used across almost all of my sites was query-string.
query-string is a super useful package for parsing URL parameters, but it now has a native replacement, URLSearchParams. It can be used both in node and the browser.
One of the reasons to remove query-string is because of the continual error:
npm WARN deprecated querystring. The querystring API is considered Legacy. new code should use the URLSearchParams API instead.
The following functions should help with removing this package from your codebase. Here is how to replace `query-string` with URLSearchParams
:
Stringify URL Params with URLSearchParams
I previously used query-string
to take an object of arrays, and turn into a comma separated URL. The commas within the URL were not encoded.
This turned out to be a little bit trickier with URLSearchParams
.
What used to be:
queryString.stringify(params, {
arrayFormat: 'comma',
})
Is now a bit longer:
export const stringifyParams = (params) => {
// Set up a new URLSearchParams object.
const searchParams = new URLSearchParams()
// Loop through the params object and append each key/value
// pair to the URLSearchParams object.
for (const [key, value] of Object.entries(params)) {
searchParams.append(key, value)
// If a key does not have a value, delete it.
if (!searchParams.get(key)) {
searchParams.delete(key)
}
}
// Convert the URLSearchParams object to a string.
const searchParamsString = searchParams.toString()
// Replace the encoded commas with commas.
const decodedSearchParamsString = searchParamsString.replace(/%2C/g, ',')
return decodedSearchParamsString
}
Parsing with URLStringParams
Parsing turned out to be a little easier.
What used to be:
queryString.parse(location.search, { arrayFormat: 'comma' })
Is now the following:
export const parseParams = (string) => {
// Set up a new URLSearchParams object using the string.
const params = new URLSearchParams(string)
// Get an iterator for the URLSearchParams object.
const entries = params.entries()
const result = {}
// Loop through the URLSearchParams object and add each key/value
for (const [key, value] of entries) {
// Split comma-separated values into an array.
if (value.includes(',')) {
result[key] = value.split(',')
} else {
result[key] = [value]
}
// If a key does not have a value, delete it.
if (!value) {
delete result[key]
}
}
return result
}
These functions can be added to a util file within your JS project and imported when needed.
Hopefully this helped you, and if you have any questions you can reach me at: @robertmars