How to Add media.net Advert Scripts to a React Website
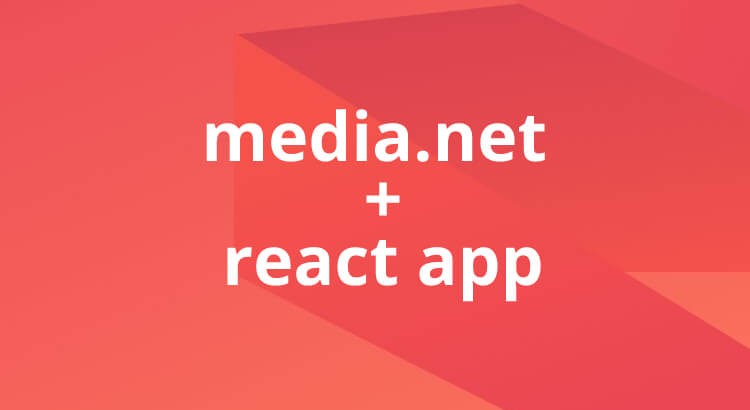
I was recently asked to add a media.net ad script onto a clients React based website, as they were moving from one ad provider to another.
My concern was media.net needs a script adding to the website, which then injects its own code. Then once this is complete it also requires individual scripts in each location that an advert is to be shown.
Media.net do not provide a React package themselves to help publishers add the script (missed opportunity I feel). So there are a number of suggested solutions online. After trying several, this is the solution I found works the best (altered to my needs).
Solution
Adding the main media.net JS script to the head
Firstly to add the main body script to a site we need to use Helmet from ‘react-helmet’ Like so:
import React from "react";
import { Helmet } from "react-helmet";
const myReactPage = () => (
<div className="application">
<Helmet>
<script type="text/javascript">
window._mNHandle = window._mNHandle || {}; window._mNHandle.queue =
window._mNHandle.queue || []; medianet_versionId = "YOUR VERSION ID";
</script>
<script
src="https://contextual.media.net/dmedianet.js?cid=YOUR CUSTOMER ID"
async="async"
></script>
</Helmet>
{/* Rest of page */}
</div>
);
(Make sure to update the script to use your IDs.)
Adding media.net ads to each location
Then to allow us to simply re-use the Ad component throughout the site easily, make a component for where we want the media.net ads to show up.
import React, { useEffect } from "react";
const MediaNetAd = ({ id, size }) => {
useEffect(() => {
if (typeof window !== "undefined") {
try {
window._mNHandle.queue.push(function () {
window._mNDetails.loadTag(id, size, id);
});
} catch (error) {}
}
}, [id, size]);
return (
<div className="mediaAdWrapper">
<div id={id} />
</div>
);
};
export default MediaNetAd;
I added a wrapper around the div that holds the id. This allow me to control the spacing between the adverts and my content using CSS.
This component is then used like this:
const PageOfApp = () => (
<div className="content">
{/* Something here */}
<MediaNetAd id="YOUR ID" size="300x250" />
{/* Something here */}
</div>
);
Depending on how much control you wanted, you could move the ID into the component so you only need to pass in the size prop.
I hope this helps!