How to use componentWillUnmount in Functional Components in React
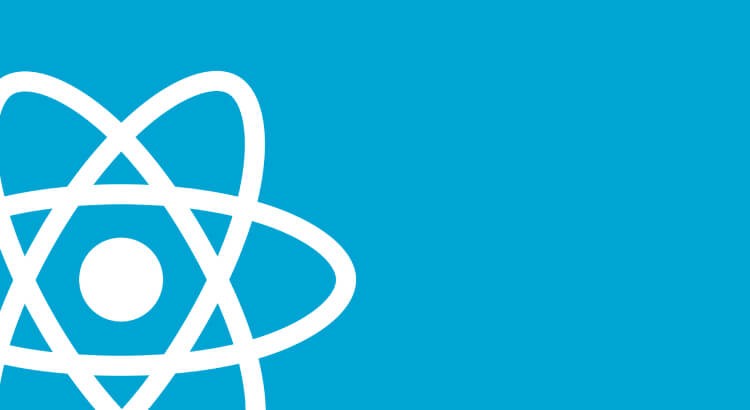
This post contain affiliate links to Udemy courses, meaning when you click the links and make a purchase, I receive a small commission. I only recommend courses that I believe support the content, and it helps maintain the site.
Functional components are far more efficient than class based components. Less code is needed to be written to achieve the same goal.
This post explains how functional components can carry out life-cycle events without needing to be turned into a class based component. In a nutshell, how to properly use componentWillMount and componentWillUnmount in a functional component with a useEffect in React.
Table of Contents
- How to manage componentWillMount in useEffect
- How to manage componentWillUnmount with useEffect
- Both componentWillMount and componentWillUnmount in useEffect
How to manage componentWillMount in useEffect
Turns out everything can be managed through useEffect.
useEffect
can be used to manage API calls, as well as implementing componentWillMount
, and componentWillUnmount
. Both are very similar!
To understand how to use componentWillUnmount
in functional components, first we need to look at how the component manages mounting with useEffect
. Shown in the example below:
import React, { useEffect } from 'react';
const ComponentExample => () => {
useEffect( () => {
// componentwillmount in functional component.
// Anything in here is fired on component mount.
}, []);
}
If we pass an empty array as the second argument, it tells the useEffect
function to fire on component render (componentWillMount
). This is the only time it will fire.
With this in mind, how can we alter the code to work with componentWillUnmount
?
How to manage componentWillUnmount with useEffect
A common question is ‘what does the return in a useEffect
do?’ A useEffect
return is called on unmount, meaning it can be used to clear setTimeouts
, remove eventListeners
etc.
How to use return in a useEffect
To use componentWillUnmount
within a useEffect
, first add a return function to the useEffect
. This is triggered when a component unmounts from the DOM. The example below shows how to unmount in a React functional component:
import React, { useEffect } from 'react';
const ComponentExample => () => {
useEffect(() => {
return () => {
// componentwillunmount in functional component.
// Anything in here is fired on component unmount.
}
}, [])
}
Combining both componentDidMount and componentWillUnmount
It is possible to combine both componentDidMount
, and componentWillUnmount
in the same useEffect
function call. Meaning handling a component will unmount, and component will mount call in the same snippet. Dramatically reducing the amount of code needed to manage both life-cycle events.
The example below shows componentDidMount
and componentWillUnmount
both being used within a functional components.
import React, { useEffect } from 'react';
const ComponentExample => () => {
useEffect(() => {
// Anything in here is fired on component mount.
return () => {
// Anything in here is fired on component unmount.
}
}, [])
}
For more React hints and tips take a look at the React Category.
I would love to know your thoughts on the above. Please let me know at @robertmars