Using WordPress Shortcodes with Next JS
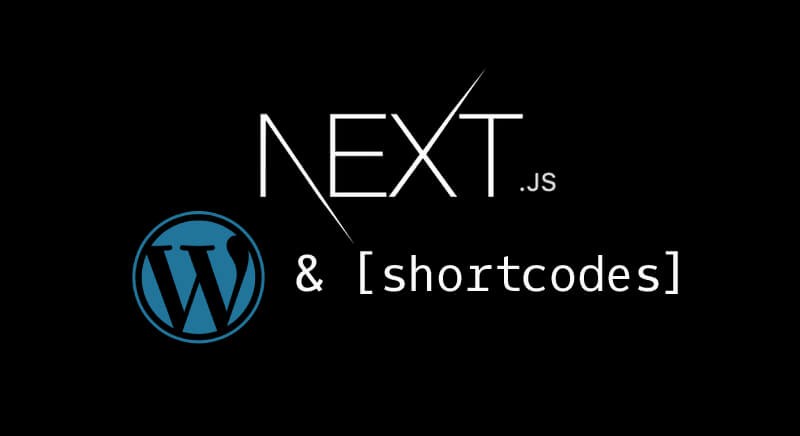
This post contain affiliate links to Udemy courses, meaning when you click the links and make a purchase, I receive a small commission. I only recommend courses that I believe support the content, and it helps maintain the site.
WordPress works really well as a headless CMS for NextJS. For most things it is relatively plug and play. However something that I initially struggled with was how to implement Shortcodes within WordPress to render something specific in NextJS.
Examples of this could be forms that need to be places in specific places within an articles content. Or advert blocks that you want to position in exact positions. Using the WordPress shortcode style of [advert-block]
or [contact-form]
you can make sure that your NextJS React components are rendered in the correct place.
The Solution to WordPress NextJS Shortcodes
The solution is to write some custom code to work alongside the package html-react-parser.
html-react-parse makes it easier to loop through your content nodes passed in from WordPress and find a particular node within a HTML block. This node can then be manipulated, changed, or removed.
Here is an example of the solution. The shortcode used within the WordPress content is [newsletter-form].
import React from "react";
import parse from "html-react-parser";
// An example component so we have something to return.
const AdvertBlockComponent = () => <div>Your custom ad code goes here</div>;
// Util function to handle the shortcode find/replace.
const handleShortcodes = (node) => {
// It seems all shortcodes are P tags.
// So we check that the node has a type, and that that type
// is a tag.
if (node.type && node.type === "tag") {
// If this tag has children, and it is the first child.
// This may cause issues, but I have not had issues with it.
// The first array is usually a shortcode is the first and
// only child of a tag node.
const shortcode = node.children[0]?.data;
// If we find the shortcode string, replace it with
// our component.
if (shortcode === "[advert-block]") {
return <AdvertBlockComponent />;
}
}
// If nothing then return original node
return node;
};
// This is the component you would call in your page, and pass in the raw content HTML.
const Wysiwyg = ({ children }) => {
const reactElements = parse(children || "", {
replace: handleShortcodes,
});
return <div>{reactElements}</div>;
};
Hopefully this article has helped, and if you have any questions you can reach me at: @robertmars