Check React Component Props are Passed to Child in Jest Unit Test
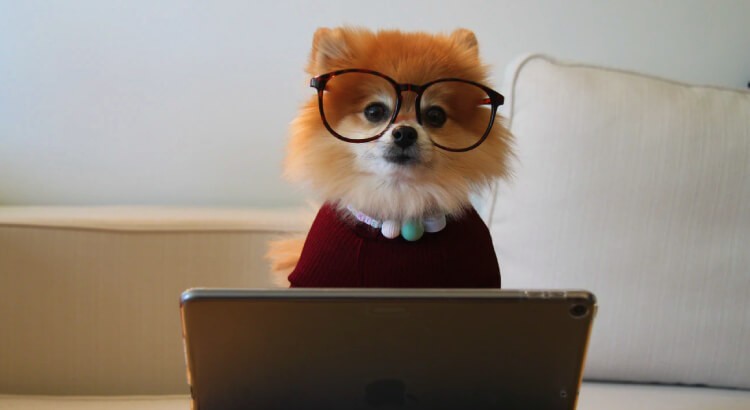
This post contain affiliate links to Udemy courses, meaning when you click the links and make a purchase, I receive a small commission. I only recommend courses that I believe support the content, and it helps maintain the site.
This short article aims to explain how to unit test a parent components logic, and check that a child component is passed the correct props. A quick how to on using Jest to check if props are passed to a child component. I initially struggled to wrap my head around how can I test if a props is passed to a child. I.e. How to mock a child component in Jest – hopefully this helps you!
The mindset behind this is that the child components have already been unit tested themselves, and do not need to be included in this particular test. Breaking it down to its simplest form, the only purpose of the test is to check that the correct arguments are passed to the child function. Not only does this simplify the testing, but it also helps reduce the appeal to nest the tests as the functionality is more effectively split up.
BTW, I appreciate the image banner is not particularly relevant, but finding interesting ‘react unit test passing props check’ images is a long and daunting task… And the dog is cool AF.
If you are looking to only mock the React component and not check the components props, I would recommend looking at the article on How To Mock A React Component In Jest.
Example
This example is purely to show how to verify that a React components props are passed in a Jest unit test. There are two components, a ParentComponent and a ChildComponent. The ParentComponent can take a prop of open
, and data
. When open
is set to true the child component is shown, and the data is passed into it.
So – How to test if a component is rendered with the right props using React Testing Library.
For this example imagine that the ChildComponent is stored in the same folder as the ParentComponent.
ParentComponent
import React from "react";
import ChildComponent from "./ChildComponent";
const ParentComponent = ({ open, data }) => (
<>
<p>Some content to render</p>
{open && <ChildComponent open={open} data={data} />}
</>
);
export default ParentComponent;
The Complete Test
Scroll down for a broken down explaination.
import React from "react";
import { render } from "@testing-library/react";
import ParentComponent from "./ParentComponent";
const mockChildComponent = jest.fn();
jest.mock("./ChildComponent", () => (props) => {
mockChildComponent(props);
return <mock-childComponent />;
});
test("If ParentComponent is passed open and has data, ChildComponent is called with prop open and data", () => {
render(<ParentComponent open data="some data" />);
expect(mockChildComponent).toHaveBeenCalledWith(
expect.objectContaining({
open: true,
data: "some data",
})
);
});
test("If ParentComponent is not passed open, ChildComponent is not called", () => {
render(<ParentComponent />);
expect(mockChildComponent).not.toHaveBeenCalled();
});
Break Down
Setting Up the Mocked ChildComponent
The first thing that must happen is the component that is to be tested needs to be mocked. This happens outside of any test
or it
blocks.
// Set up a Jest mock function to check any props.
const mockChildComponent = jest.fn();
// Mock the child component file, and grab all props passed to it
// and pass them to the Jest mock function so it can listen.
jest.mock("./ChildComponent", () => (props) => {
mockChildComponent(props);
// Return something for React to render. I like creating
// an element to pass through as it
// helps with visually debugging if needed.
return <mock-childComponent />;
});
Testing The ParentComponent is Setting Props Correctly
Then the test block can be set up and the ParentComponent rendered with React Testing Library. The props of the ParentComponent should be set (or not, depending on the test) and then an expect
is put in place for checking the passed props.
test("If ParentComponent is passed open and has data, ChildComponent is called with prop open and data", () => {
// Render the ParentComponent with the props wanting to
// be tested.
render(<ParentComponent open data="some data" />);
// Check that the Jest mock function is called with an object.
// Use 'expect.objectContaining' to make sure any other default
// React props are ignored.
expect(mockChildComponent).toHaveBeenCalledWith(
expect.objectContaining({
open: true,
data: "some data",
})
);
});
This way of checking if the correct props have been set has massively simplified my unit testing. I quite often need to Jest Test that React component has passed props correctly, and this layout is quick and clean.
Take a look at the article on testing with Jest and React Testing Library for a basic overview of unit testing.
For more React and Jest hints and tips take a look at the React Category, and Jest Testing Category!