How To Mock A React Component In Jest
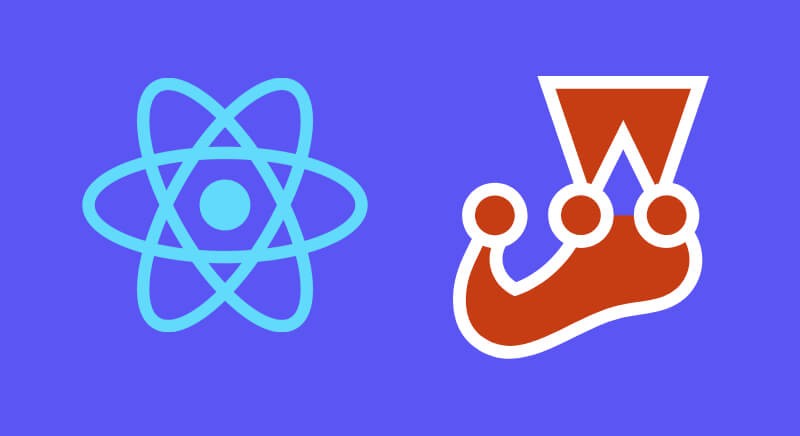
This post contain affiliate links to Udemy courses, meaning when you click the links and make a purchase, I receive a small commission. I only recommend courses that I believe support the content, and it helps maintain the site.
This post gives examples of how to simply mock a React component in Jest. It will cover default and named exported components.
I have previously written a piece on how to mock React components in Jest, and check to make sure that the component was passed specific props. I found that sometimes I don’t need to check the props. A lot of the time I only want to make sure the component has been rendered on the page. I want to mock it and expect it to be called.
The understanding is that the component itself will either be tested by a third party so it does not need testing, or has already been tested in a different test file. By mocking the imported component we can reduce the complexity of a test – breaking it down to its simplest form.
Example
There are two components, TopLevelComponent
and Modal
. The TopLevelComponent
can take a prop of open. When open is set to true the Modal
is shown. The test does not want to have to mock any of the Modal
internals. We just want to test if the Modal
is rendered or not.
import React from "react";
import Modal from "./Modal";
const TopLevelComponent = ({ open }) => (
<>
<p>Some other content to render...</p>
{open && <Modal />}
</>
);
export default TopLevelComponent;
[support-block]
The Complete Test
To mock a React component within Jest you should use the `jest.mock` function. The file that exports the specific component is mocked and replaced with a custom implementation. Since a component is essentially a function, the mock should also return a function. Leading on from the example above, the tests would look like so:
import React from "react";
import { render } from "@testing-library/react";
import TopLevelComponent from "./TopLevelComponent";
jest.mock("./Modal", () => () => {
return <mock-modal data-testid="modal"/>;
});
test("If TopLevelComponent is passed the open prop Modal is rendered", () => {
const { queryByTestId } = render(<TopLevelComponent open />);
expect( queryByTestId("modal") ).toBe(true)
});
test("If TopLevelComponent is not passed the open prop Modal is not rendered", () => {
const { queryByTestId } = render(<TopLevelComponent />);
expect( queryByTestId("modal") ).toBe(false);
});
But My Component is a Named Export
The above example uses a default exported function. It is the main item to be exported from its file. However, how do we mock the component when it is a named export? i.e – But how do you mock a React component that is the named export of a file?
import React from "react";
import { Modal } from "./ManyModals";
const TopLevelComponent = ({ open }) => (
<>
<p>Some other content to render...</p>
{open && <Modal />}
</>
);
export default TopLevelComponent;
There are several things to consider, is it an ES6 module or not? The first example below example is not, and is slightly simpler:
jest.mock("./ManyModals", () => ({
Modal: () => {
return <mock-modal data-testid="modal"/>;
},
}));
However if you are working with ES6 Modules then you will need to do the following:
jest.mock("./ManyModals", () => ({
__esModule: true,
Modal: () => {
return <mock-modal data-testid="modal"/>;
},
}));
This way of checking if the correct props have been set has massively simplified my unit testing. I quite often need to Jest Test that React component has passed props correctly with React Testing Library, and this way of handling them is quick and clean.
For more about importing and mocking ES6 Modules take a look at this article.
What About Testing the Props?
I have written another piece on that over at: Check React Component Props are Passed to Child in Jest Unit Test. That shows how to mock React components with a little more complexity.
For more React and Jest hints and tips take a look at the React Category, and Jest Testing Category!
Take a look at the article on testing with Jest and React Testing Library for a basic overview of unit testing.
Hopefully this article has helped, and if you have any questions you can reach me at: @robertmars